Machine-to-machine authentication often starts with static API keys. They're easy to implement and get the job done when you're building early-stage APIs. But when you're preparing to support large-scale integrations, especially under evolving standards like MCP, static credentials quickly become a liability.
In 2025, OAuth 2.1 becomes mandatory for MCP servers. If you’ve built your current auth system around API keys, this guide will help you migrate with minimal friction. We’ll walk through the key differences, explain how OAuth 2.1 improves security and traceability, and give you practical examples using Scalekit to streamline the process.
By the end of this guide, your APIs will be secured with short-lived, scoped tokens issued through a standards-compliant authorization server, without needing to build everything from scratch.
Why API keys don’t cut it anymore
API keys are static tokens passed in headers. That’s their strength and their biggest weakness. Once issued, they stay valid forever, unless someone revokes or rotates them manually.
This might work when you control both client and server. However, in modern SaaS systems with third-party integrations, multiple environments, and compliance requirements, API keys expose too much surface area. You can’t limit what the client can access. You can’t trace who made what request. And once the key is compromised, you have no safety net.
Take a common example: an internal order service making calls to an nventory API. You might authorize the request by passing an API key in a custom header. Simple. But there’s no visibility. No token expiration. No way to prove which team or tenant made the call. As your system grows, this approach breaks down.
OAuth 2.1 secures machine-to-machine communication
OAuth 2.1 introduces a better way to handle service-to-service authentication.
Instead of passing static keys, a client first requests an access token from an authorization server. The request uses machine credentials, a client_id, and client_secret. If the credentials are valid, the server issues a time-bound token. That token is then sent as a bearer token in the API request.
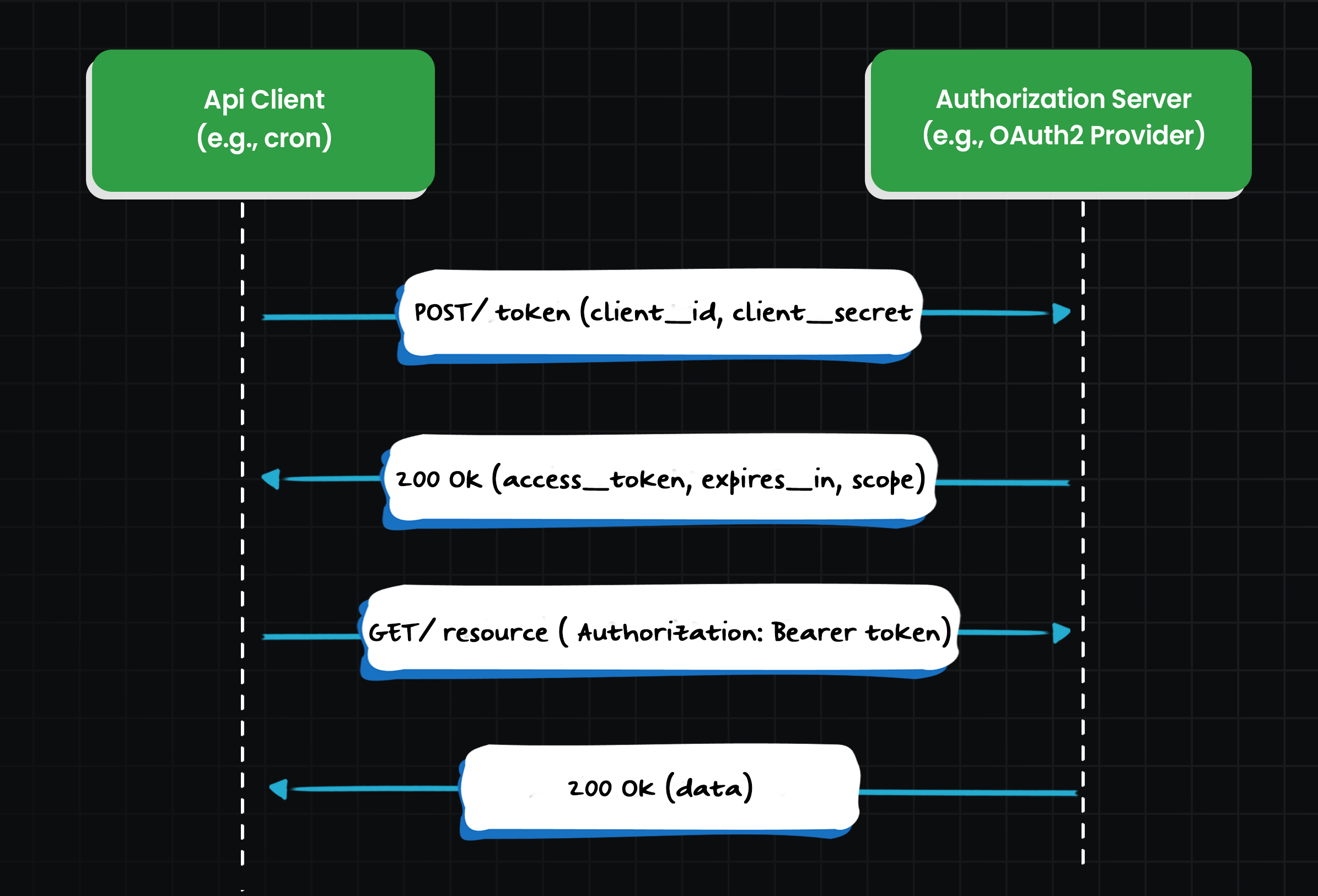
Authorization: Bearer eyJhbGciOi...
This flow is called the Client Credentials Grant. It doesn't require user interaction and is purpose-built for backend services talking to each other.
Here’s what it brings to the table:
- Tokens are short-lived and scoped, reducing impact if compromised.
- Clients can be uniquely identified and isolated.
- Audit logs can trace every token request and usage.
- Rotation becomes easier, generate new credentials, and revoke old ones.
- Most importantly, it aligns with existing enterprise and platform standards.
MCP's latest spec mandates OAuth 2.1 for a reason. It’s more secure, more scalable, and far easier to govern across tenants and environments.
Migrating to OAuth 2.1 takes effort, but it’s worth it
Switching from API keys to OAuth 2.1 is not just about changing how tokens are passed. It requires you to rethink how your systems authenticate clients, issue credentials, and validate incoming requests.
You’ll need an authorization server to issue tokens. Your clients must know how to request and cache those tokens. And your APIs must validate every incoming token before serving any data.
This isn’t a small lift. But the payoff is clear: you gain control over who accesses what, when, and for how long. You eliminate the need for manual key rotation. You reduce your blast radius in the event of a leak. You also align your platform with the same practices used by Google service accounts, OpenAI tool integrations, and enterprise-grade APIs.
If your team is managing MCP servers or planning to onboard enterprise clients, OAuth 2.1 is no longer optional. It’s the new baseline.
Simplify token management with Scalekit
You can build your own authorization server using open-source tools like Keycloak or ORY Hydra. But that means handling secure client registration, managing token issuance, exposing JWKS endpoints, and maintaining uptime.
Scalekit abstracts all of this.
It acts as a managed authorization layer for machine-to-machine authentication. Clients request tokens from Scalekit. Your APIs validate those tokens using standard JWT verification. And everything follows the OAuth 2.1 spec, from scoped access to tenant-aware claims.
Instead of managing key infrastructure, you focus on what matters: issuing credentials to your clients and securing your APIs.
Here’s how it works:
- You register your client machines with Scalekit.
- Clients use their credentials to request scoped access tokens.
- APIs validate those tokens using Scalekit’s JWKS URL.
- You enforce org-level isolation with claims like org_id.
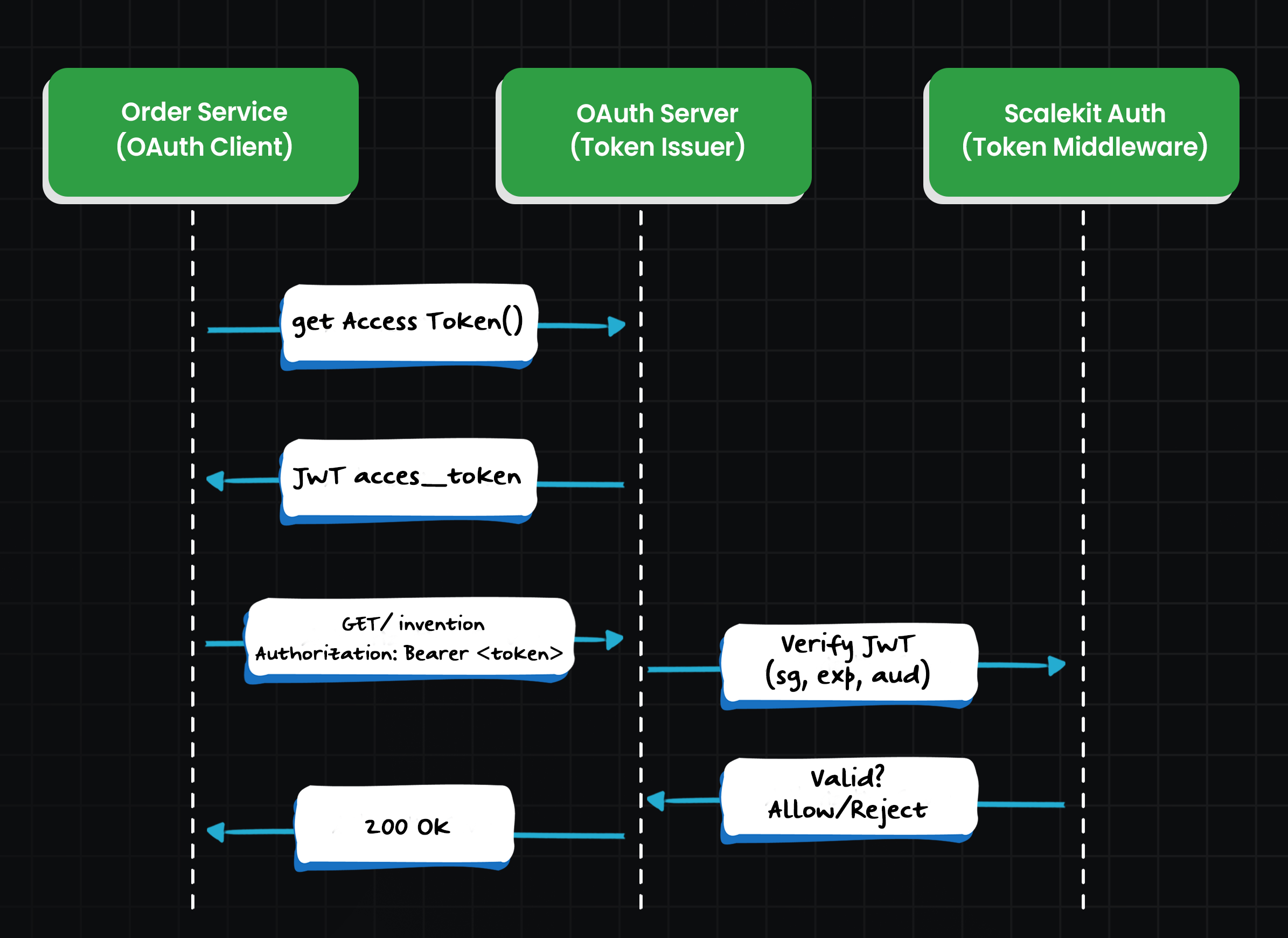
Let’s walk through how to set this up, step by step.
Step 1: Register your machine client in Scalekit
Before a service can authenticate using OAuth 2.1, it needs credentials. This means creating a client identity that can request tokens from the authorization server.
In Scalekit, this is a simple API call or dashboard action.
You’ll define:
- A client name (e.g., order-service)
- It's allowed scopes (e.g., read:inventory)
- The organization it belongs to (for tenant isolation)
- The callback URL if you ever expand to flows beyond M2M
Once registered, Scalekit returns two values:
- client_id: Public identifier
- client_secret: Private credential (store this securely)
You’ll use these to request tokens in the next step.
Step 2: Exchange credentials for an access token
In your client application, you’ll use the client_id and client_secret to get a bearer token from Scalekit’s token endpoint.
Here’s an example in Node.js:
The response includes an access token, its type (Bearer), and its expiration. Most tokens are valid for 3600 seconds (1 hour), but always check the expires_in field.
You’ll cache this token in memory or a short-lived store, and re-request it only after expiration. Avoid requesting a new token on every API call.
Step 3: Call your API using the Bearer token
Once you have a token, include it in the Authorization header when making API calls:
That’s it from the client’s side. Your service is now sending scoped, time-bound, OAuth-compliant requests.
In the next step, we’ll shift focus to the API server, where the token needs to be validated before granting access.
Step 4: Validate tokens on your API server
When your API receives a request with a Bearer token, it must validate that token before serving data. This step is non-negotiable, especially if you’re building a compliant MCP server.
Token validation has three parts:
- Verify the signature
- Check the token’s metadata
- Enforce any custom claims
Scalekit issues JWTs signed with asymmetric keys. That means your server can validate tokens without needing a shared secret, just the public key, which is available via JWKS.
Example: JWT middleware in Node.js (Express)
What this validates
- Signature: Ensures token wasn’t tampered with
- Issuer: Must be Scalekit’s auth server
- Audience: Must match your API
- Expiration: Token must still be valid
- org_id (optional): Confirms tenant-level access
This is the core of OAuth 2.1 token validation. Once in place, your API can confidently handle requests from trusted clients, with no more static secrets and no more guessing who’s calling.
Phase out API keys with a safe rollout plan
You don’t have to flip the switch overnight. A phased migration lets you move services to OAuth 2.1 gradually, without breaking existing integrations or rushing clients.
Here’s how to manage the transition step-by-step:
1. Inventory existing API key usage
Start by mapping out where API keys are being used. This includes:
- Internal services calling other internal APIs
- Customer SDKs or partner integrations
- CI/CD tools and backend automation scripts
Look for hardcoded keys, references in environment variables, and logging metadata. Try to link each key to the client or service that owns it.
2. Define scopes and isolate tenants
For each client, ask: What do they actually need access to? Don’t issue broad tokens that grant more than required.
- Create fine-grained scopes like read:inventory, write:orders, or admin:billing.
- For multi-tenant systems, enforce tenant separation using claims like org_id.
This gives you precise control and reduces the blast radius in case of a leak.
3. Add dual support in APIs
Your API endpoints should accept both API keys and OAuth 2.1 tokens during the transition.
In Express, for example:
This gives you flexibility while slowly migrating clients one by one.
4. Migrate clients and monitor usage
Start with internal services you control. Then move to trusted partners or customers with direct access.
Use feature flags or environment config to toggle between auth modes per client. This avoids big bang releases.
Scalekit provides logs and dashboards to help you track which clients are still using keys vs. tokens.
5. Set a clear sunset date
Once a majority of clients have migrated, communicate a hard cutoff for API key support.
Give at least 30–60 days of notice. Show token-based examples. Offer fallback support during rollout.
Once the date passes, remove the API key logic and enforce token-only access.
Securing internal service communication with OAuth 2.1
Let’s say you have two services inside your stack:
- Order Service: responsible for creating and managing orders
- Inventory Service: keeps track of product stock
In your current setup, the Order Service calls the Inventory API using a static API key:
This works, but the key is static, has no scope, and offers no visibility. If leaked, anyone could query your inventory.
Here’s how the same flow works after migrating to OAuth 2.1 using Scalekit.
Step 1: Order Service fetches a token
The Order Service authenticates itself using its client credentials:
This returns a short-lived bearer token tied to the service and its allowed scope.
Step 2: Order Service calls Inventory API
The service includes the bearer token in the request:
This token is only valid for a specific duration (say, 1 hour) and only for actions like read:inventory.
Step 3: Inventory API validates the token
The Inventory Service uses Scalekit’s JWKS endpoint to verify the token:
- Confirms the signature is valid
- Checks aud and iss match expected values
- Confirms the token has not expired
- Validates that the scope includes read:inventory
- Optionally, checks org_id to isolate tenants
Only if all checks pass is the request fulfilled.
With this setup, you’ve:
- Replaced a static key with a rotating token
- Scoped down access per service
- Added traceability to every request
- Reduced the risk from credential leaks
This is what MCP-compliant, machine-to-machine security looks like.
Common issues when implementing OAuth 2.1 for M2M
OAuth 2.1 improves security, but only if implemented fully and correctly. These are the areas where most teams trip up, and what to watch out for.
Skipping full token validation
It’s not enough to check if a token exists or is well-formed. Your API must validate:
- Signature: Using the public key from the JWKS endpoint
- Issuer (iss): Should match the expected authorization server
- Audience (aud): Should match your API
- Expiration (exp): Must still be valid
- Scope: Must include the required permission
- Tenant claim (org_id): If multi-tenant, must match the requesting org
Ignoring any of these checks weakens the entire flow.
Using overly broad scopes
Giving every client admin:all access defeats the purpose of OAuth. Define fine-grained scopes and only assign what each client truly needs.
This limits damage if a client is compromised and helps enforce least privilege.
Storing client_secret insecurely
Your client_secret is sensitive. Never check it into version control or expose it in logs.
Best practices:
- Load it from a secure secrets manager
- Scope environment variables tightly
- Rotate periodically and monitor usage
Over-requesting tokens
Some services call the token endpoint on every API request. This adds unnecessary latency and load.
Instead, cache the token in memory or a lightweight store and reuse it until it expires. Then fetch a new one.
Expecting refresh tokens in Client Credentials flow
Standard OAuth 2.1 client credentials do not return a refresh token. The token expires, and you request a new one. This is by design.
If you need to refresh logic, you’re probably using the wrong grant type.
Migrating to OAuth 2.1 future-proofs your API security
Migrating from API keys to OAuth 2.1 is a crucial step in enhancing the security and scalability of your machine-to-machine communication. As you’ve seen throughout this guide, moving to OAuth 2.1 brings significant improvements over the limitations of static API keys. You’ve learned how to implement OAuth 2.1 for your MCP servers, leveraging Scalekit to handle token issuance and validation seamlessly. This transition allows you to issue scoped, short-lived tokens and implement robust security practices that align with industry standards.
By adopting OAuth 2.1, you future-proof your API security, ensuring it scales with modern architecture, compliance requirements, and customer needs. This move not only meets MCP server specifications but also strengthens the overall security posture of your platform, allowing for better control over access and permissions across various environments and tenants.
As you begin the migration process, remember that transitioning gradually with dual support for both API keys and OAuth 2.1 will ease the shift. Scalekit provides a straightforward way to manage this process, ensuring your APIs remain secure while facilitating smooth adoption across all clients. If you’re ready to secure your M2M communications with OAuth 2.1 and take your API security to the next level, get started with Scalekit today.
FAQs
What’s the difference between API keys and OAuth 2.1 tokens?
API keys are static credentials; once issued, they stay valid until revoked. OAuth 2.1 tokens are short-lived, scoped, and signed, making them more secure, traceable, and easier to manage across distributed systems. Here's a deep-dive into the topic.
Do I need to rotate OAuth 2.1 tokens?
No. Tokens in the Client Credentials flow are short-lived by design. Once expired, the client simply requests a new one. There’s no refresh token involved.
Can I use Scalekit for machine-to-machine authentication?
Yes. Scalekit can handle M2M authentication, with support for multi-tenant token claims like org_id, scoped tokens, and full OAuth 2.1 compliance. It abstracts token issuance and validation, so you don’t need to host or maintain your own auth server.
How do I test this in development?
Scalekit offers test organizations, default scopes, and even an IdP simulator if you extend into user-auth flows later. You can spin up the full token lifecycle in your local setup without needing to register clients in a production environment.
What happens to existing API key clients during migration?
You can support both API key and OAuth 2.1 flows in parallel. Add conditional logic in your middleware to handle both types of authorization headers. Once all clients are migrated, phase out API key validation safely.